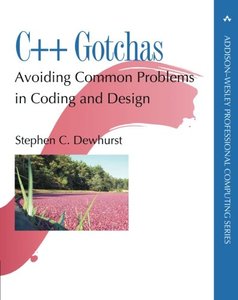
C++ Gotchas: Avoiding Common Problems in Coding and Design (Paperback)
內容描述
C++ Gotchas is a guide to avoiding and correcting ninety-nine of the
most common, destructive, and interesting C++ design and programming errors.
Students will get a look inside look at the more subtle C++ features and
programming techniques.
This book discusses basic errors present in almost all C++ code, as well as
complex mistakes in syntax, preprocessing, conversions, initialization, memory
and resource management, polymorphism, class design, and hierarchy design. Each
error and its repercussions are explained in context, and the resolution of each
problem is detailed and demonstrated.
Author Stephen Dewhurst supplies students with idioms and design patterns
that can be used to generate customized solutions for common problems. students
will also learn more about commonly misunderstood features of C++ used in
advanced programming and design. A companion Web site, located at
http://www.semantics.org, includes detailed code samples from the book.
Table of Contents
Preface. Acknowledgments. 1. Basics.
Gotcha #1: Excessive Commenting. Gotcha #2:
Magic Numbers. Gotcha #3: Global Variables. Gotcha #4: Failure to
Distinguish Overloading from Default Initialization. Gotcha #5:
Misunderstanding References. Gotcha #6: Misunderstanding Const. Gotcha
7: Ignorance of Base Language Subtleties. Gotcha #8: Failure to Distinguish
Access and Visibility. Gotcha #9: Using Bad Language. Gotcha #10:
Ignorance of Idiom. Gotcha #11: Unnecessary Cleverness. Gotcha #12:
Adolescent Behavior.
- Syntax.
Gotcha #13: Array/Initializer Confusion.
Gotcha #14: Evaluation Order Indecision. Gotcha #15: Precedence
Problems. Gotcha #16: for Statement Debacle. Gotcha #17: Maximal Munch
Problems. Gotcha #18: Creative Declaration-Specifier Ordering. Gotcha
#19: Function/Object Ambiguity. Gotcha #20: Migrating Type-Qualifiers.
Gotcha #21: Self Initialization. Gotcha #22: Static and Extern Types.
Gotcha #23: Operator Function Lookup Anomaly. Gotcha #24: Operator —
Subtleties. - The Preprocessor.
Gotcha #25: #define Literals. Gotcha #26:
#define Pseudofunctions. Gotcha #27: Overuse of #if. Gotcha #28: Side
Effects in Assertions. Conversions.
Gotcha #29: Converting Through void *.
Gotcha #30: Slicing. Gotcha #31: Misunderstanding Pointer-to-Const
Conversion. Gotcha #32: Misunderstanding Pointer-to-Pointer-to-Const
Conversion. Gotcha #33: Misunderstanding Pointer-to-Pointer-to-Base
Conversion. Gotcha #34: Pointer-to-MultiDimensional Array Problems.
Gotcha #35: Unchecked Downcasting. Gotcha #36: Misusing Conversion
Operators. Gotcha #37: Unintended Constructor Conversion. Gotcha #38:
Casting Under Multiple Inheritance. Gotcha #39: Casting Incomplete Types.
Gotcha #40: Old-Style Casts. Gotcha #41: Static Casts. Gotcha #42:
Temporary Initialization of Formal Arguments. Gotcha #43: Temporary
Lifetime. Gotcha #44: References and Temporaries. Gotcha #45: Ambiguity
Failure of dynamic_cast. Gotcha #46: Misunderstanding Contravariance.Initialization.
Gotcha #47: Assignment/Initialization Confusion.
Gotcha #48: Improperly Scoped Variables. Gotcha #49: Failure to
Appreciate C++'s Fixation on Copy Operations. Gotcha #50: Bitwise Copy of
Class Objects. Gotcha #51: Confusing Initialization and Assignment in
Constructors. Gotcha #52: Inconsistent Ordering of the Member Initialization
List. Gotcha #53: Virtual Base Default Initialization. Gotcha #54: Copy
Constructor Base Initialization. Gotcha #55: Runtime Static Initialization
Order. Gotcha #56: Direct versus Copy Initialization. Gotcha #57: Direct
Argument Initialization. Gotcha #58: Ignorance of the Return Value
Optimizations. Gotcha #59: Initializing a Static Member in a Constructor.Memory and Resource Management.
Gotcha #60: Failure to Distinguish Scalar and
Array Allocation. Gotcha #61: Checking for Allocation Failure. Gotcha
#62: Replacing Global New and Delete. Gotcha #63: Confusing Scope and
Activation of Member new and delete. Gotcha #64: Throwing String Literals.
Gotcha #65: Improper Exception Mechanics. Gotcha #66: Abusing Local
Addresses. Gotcha #67: Failure to Employ Resource Acquisition Is
Initialization. Gotcha #68: Improper Use of auto_ptr.- Polymorphism.
Gotcha #69: Type Codes. Gotcha #70:
Nonvirtual Base Class Destructor. Gotcha #71: Hiding Nonvirtual Functions.
Gotcha #72: Making Template Methods Too Flexible. Gotcha #73:
Overloading Virtual Functions. Gotcha #74: Virtual Functions with Default
Argument Initializers. Gotcha #75: Calling Virtual Functions in Constructors
and Destructors. Gotcha #76: Vir